How to develop Scalable Programs as a Developer By Gustavo Woltmann
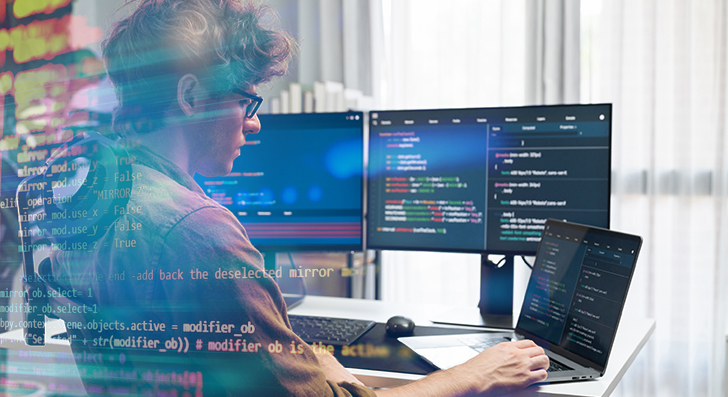
Scalability usually means your software can take care of expansion—a lot more customers, more facts, plus much more targeted traffic—without having breaking. As a developer, developing with scalability in your mind saves time and stress later on. Right here’s a transparent and useful guide that may help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of one's system from the start. Many apps are unsuccessful whenever they grow rapidly because the initial structure can’t manage the extra load. To be a developer, you should Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to be versatile. Steer clear of monolithic codebases the place all the things is tightly connected. Alternatively, use modular design or microservices. These patterns split your application into lesser, impartial pieces. Every module or provider can scale By itself without affecting The entire process.
Also, give thought to your database from working day a person. Will it require to deal with 1,000,000 people or simply just a hundred? Choose the correct variety—relational or NoSQL—according to how your knowledge will develop. Program for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional essential level is in order to avoid hardcoding assumptions. Don’t write code that only works below existing situations. Think of what would come about When your consumer base doubled tomorrow. Would your app crash? Would the databases slow down?
Use design and style patterns that assistance scaling, like message queues or event-driven systems. These assistance your application handle more requests without getting overloaded.
When you Establish with scalability in your mind, you're not just getting ready for success—you might be cutting down long term headaches. A perfectly-prepared technique is simpler to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the correct Database
Deciding on the suitable database is a vital Section of creating scalable applications. Not all databases are designed precisely the same, and utilizing the Improper you can sluggish you down or perhaps induce failures as your app grows.
Begin by being familiar with your knowledge. Is it remarkably structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good in shape. They are potent with associations, transactions, and regularity. Additionally they aid scaling techniques like read through replicas, indexing, and partitioning to handle additional visitors and facts.
Should your details is more versatile—like user action logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your examine and write designs. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Have you been dealing with a major create load? Take a look at databases that may take care of significant write throughput, and even function-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also good to Consider in advance. You might not need Sophisticated scaling functions now, but selecting a database that supports them signifies you received’t will need to modify afterwards.
Use indexing to speed up queries. Stay away from needless joins. Normalize or denormalize your knowledge determined by your entry designs. And generally watch databases general performance when you improve.
In short, the proper database depends on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose correctly—it’ll preserve a great deal of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, every single tiny delay provides up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct efficient logic from the beginning.
Start off by composing clean, very simple code. Prevent repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple a person performs. Keep your capabilities quick, focused, and straightforward to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too long to operate or utilizes far too much memory.
Up coming, look at your databases queries. These usually gradual items down more than the code by itself. Make sure Every single question only asks for the information you truly require. Prevent Choose *, which fetches all the things, and as a substitute choose precise fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular across huge tables.
For those who discover precisely the same data staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced functions.
Also, batch your database Developers blog operations if you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job good with 100 information may possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be smooth and responsive, even as the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more end users and a lot more website traffic. If anything goes by a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching are available. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the perform, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it might be reused speedily. When consumers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it through the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
two. Shopper-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down databases load, improves pace, and will make your app extra effective.
Use caching for things which don’t alter generally. And usually be sure your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but potent tools. Collectively, they assist your application manage additional users, remain rapid, and recover from difficulties. If you intend to mature, you will need both equally.
Use Cloud and Container Applications
To construct scalable programs, you'll need equipment that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you need them. You don’t need to acquire hardware or guess potential ability. When targeted traffic boosts, you could increase extra means with just some clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container packages your app and all the things it ought to run—code, libraries, settings—into one unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If just one section of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container applications implies you can scale rapidly, deploy simply, and Get better swiftly when problems come about. If you want your app to improve without having boundaries, begin employing these tools early. They preserve time, cut down danger, and make it easier to stay focused on constructing, not correcting.
Keep track of Almost everything
Should you don’t watch your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk Area, and response time. These let you know how your servers and companies are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Regulate how much time it's going to take for users to load pages, how often errors happen, and where they happen. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This can help you correct troubles quickly, frequently prior to users even see.
Checking can be beneficial whenever you make changes. For those who deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to serious problems.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best tools in position, you stay on top of things.
In brief, checking assists you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even compact apps have to have a powerful Basis. By designing meticulously, optimizing sensibly, and using the suitable tools, it is possible to build apps that improve smoothly without the need of breaking under pressure. Start off compact, Feel major, and build wise.